Objective
This cookbook describes how to import and link your assets in three easy steps.
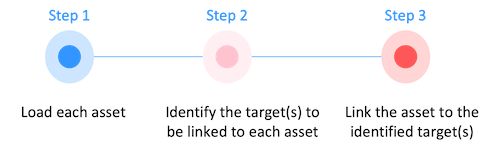
Process Overview
Importing your assets into Quable PIM is a multistep process . There are several factors that impact the quality of your data, such as dependencies and hierarchy. This is why importing your assets must be done in a specific order.
Step | Data | Description |
---|---|---|
1 | Load assets | Import each asset into the PIM. Before you can link your assets to a target (document, classification, or variant), the asset must already exist in Quable PIM. |
2 | Identify link target | Locate the target (document, classification, or variant) to which the asset will be associated. |
3 | Link assets | Link your assets to the identified target(s). |
In some cases, you only need to perform the first step:
Using Quable PIM's rule engine (Administration > System > Automatic links), you can automatically link your assets to a specific document based on the file name.
Importing assets without creating links.
Use Case
A photo studio has placed assets on a server (e.g., FTP). The name of each asset contains:
|
In order to import them, you'll need to go through the list of assets and take the following steps for each one:
|
Load Assets
Quable PIM API provides two methods to import your assets:
via URL
To create a new asset in Quable PIM, you must include the asset's code and URL.
URL Parameters | |||
Endpoint | /assets |
API v4 endpoint | |
HTTP Method | POST |
||
Request Body | |||
Required Attributes | code |
string | The unique code of the asset. |
media_url |
string | The URL to the server where the asset is located. Note: If you don't want to update the binary file, this attribute can be omitted. |
|
Accepted Attributes | active |
boolean | If False, the asset becomes inactive and is unavailable via the interface. |
{{_attribute_}} |
string | Any attribute code with its value(s) (e.g., description in the code example). | |
classification_code |
string | The DAM classification code the asset should be placed into. | |
media_original_filename |
string | The original name of the asset file. Note: If this attribute is not provided, the asset's filename will be derived from the URL. |
Example
import requests
url= "https://{{YOUR-PIM}}.quable.com.quable.com/api_1.php/assets"
payload= {
"code": "suede_shoe__jpg__01",
"media_url" : "https://domain.tld/.../suede_shoe__jpg__01.jpg",
"media_original_filename" : "suede_shoe__jpg__01.jpg",
"description" : "lorem ipsum"
}
headers= {
'Content-Type': 'application/json',
'Authorization': 'Bearer ...'
}
response= requests.request("POST", url, headers=headers, data=payload)
via Binary File
To import data from a binary file, a form based request must be used. The following example uses cURL but any other method can be used.
Request Parameters | |||
Endpoint | /assets | API v4 endpoint | |
HTTP Method | POST | -i includes the HTTP headers in the output-X identifies the method to use | |
Form Data | |||
Required Attributes | code | string | The unique code of the asset. |
file | string | The name of the binary file. |
Example
curl -i -X POST \
-H "Authorization:Bearer ..." \
-H "Content-Type:multipart/form-data" \
-F "file=@\"/assets/new/suede_shoe__jpg__01.jpg\";type=image/png;filename=\"/suede_shoe__jpg__01.jpg\"" \
-F "code=suede_shoe__jpg__01" \
'https://{{YOUR-PIM}}.quable.com/api_1.php/assets'
Identify Link Targets
Once your assets have been uploaded, you need to link them to the correct targets (documents, classifications or variants). The following sections describe how to identify document, classification, and variant targets.
Documents
URL Parameters | |||
Endpoint | /api/documents/{{id}} Note: {{id}} is the unique code of the document to update. | API v5 endpoint | |
HTTP Method | GET | ||
Query Parameters | |||
Required Attributes | id | string | The unique code of the document. |
Accepted Attributes | documentType.id | string | The unique code of the document type. |
Example
import requests
url = "https://{{YOUR-PIM}}.quable.com.quable.com/api/documents?id=suede_shoe&documentType.id=Reference"
headers = {
'Content-Type': 'application/hal+json',
'Authorization': 'Bearer ...'
}
response = requests.request("POST", url, headers=headers, data=payload)
Response Body
The response body contains all of the details for the reference document. In particular, the assetLinks array contains all of the links between the document and existing assets.You only need to check: |
| |
|
At this point, you have three options:
Scenario | Solution |
---|---|
The asset is already linked at the correct position (aka sequence). | There is nothing more to do. |
Another asset is linked at the desired position. |
|
No asset is linked or the position is incorrect. | A link with the new asset must be created. |
{
"hydra:member": [{
"id": "suede_shoe",
"active": true,
"attributes": {...},
"documentType": {
"default": false,
"id": "Reference"
},
"attributeSet": null,
"classifications": [...],
"documentLinks": [...],
"assetLinks": [{
"linkType": {
"id": "article_images_media"
},
"origin": {
"id": "suede_shoe"
},
"target": {
"id": "suede_shoe__jpg__01"
},
"sequence": 1,
"id": "e5924756-513c-4b0f-8866-8d36262f5914"
}],
"workflows": [],
"tags": [],
"completenesses": {...},
"variants": [...]
}],
"hydra:totalItems": 1,
...
}
Classifications
URL Parameters | |||
Endpoint | /api/classification/{{id}} Note: {{id}} is the unique code of the classification to update. | API v5 endpoint | |
HTTP Method | GET | ||
Query Parameters | |||
Required Attributes | id | string | The unique code of the classification. |
Variants
URL Parameters | |||
Endpoint | /api/variants/{{id}} Note: {{id}} is the unique code of the variant to update. | API v5 endpoint | |
HTTP Method | GET | ||
Query Parameters | |||
Required Attributes | id | string | The unique code of the variant. |
Link assets
You can link your assets to: | ||
|
Documents
To create a link between documents and assets, you must include the document's code, the link type's code, and define the link's mode (behavior).
URL Parameters | |||
Endpoint | /documents/{{document_code}}/links/{{link_type_code}} | API v4 endpoint | |
HTTP Method | POST | ||
Query Parameters | |||
{{document_code}} | string | The unique code of the document. | |
{{link_type_code}} | string | The unique code of the document - asset link type. | |
Request Body | |||
Required Attributes | items | array(objects) | Array of objects to link. Each object is composed of a:
|
mode | string | You must choose one of the following two behaviors:
|
Example
import requests
import json
url= "https://{{YOUR-PIM}}.quable.com.quable.com/api_1.php/documents/suede_shoe/links/videos"
payload= {
"mode" : "update", // or replace
"items": [{
"sequence" : 1,
"target_code" : "asset_video_tour_mp4"
},{
"sequence" : 8,
"target_code" : "asset_video_mini-tour_mp4"
}]
}
headers= {
'Content-Type': 'application/json',
'Authorization': 'Bearer ...'
}
response= requests.request("POST", url, headers=headers, data=json.dumps(payload))
Classifications
For complete instructions about adding links, see 5. Import links.
link_type_code
must be the unique code of a LinkType defined between classifications and assets.target_code
must be the unique code for the new asset you've created.
URL Parameters | |||
Endpoint | /classifications/{{code}}/links/{{link_type_code}} | API v4 endpoint | |
HTTP Method | POST | ||
Query Parameters | |||
{{code}} | string | The unique code of the classification. | |
{{link_type_code}} | string | The unique code of the link type between the classification and the asset. | |
Request Body | |||
Required Attributes | items | array(objects) | Array of objects to link. Each object is composed of a:
|
mode | string | You must choose one of the following two behaviors:
|
Variants
For complete instructions about adding links, see 5. Import links.
link_type_code
must be the unique code of a LinkType defined between classifications and assets.target_code
must be the unique code for the new asset you've created.
Delete Assets
It may become necessary at some point to delete an asset from Quable PIM.
To delete an asset, you must identify the asset's unique code.
URL Parameters | |||
Endpoint | /api_1/assets/{{code}} Note: {{code}} is the unique code of the asset to delete. | API v4 endpoint | |
HTTP Method | DELETE | ||
Query Parameters | |||
Required Attributes | code | string | The unique code of the asset. |
import requests
url = "https://{{YOUR-PIM}}.quable.com.quable.com/api_1.php/assets/suede_shoe__jpg__01"
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer 883ae3ca7d90f91542227dc8d0d38b46'
}
response = requests.request("DELETE", url, headers=headers)